Java is a powerful and flexible computer language, and knowing its more advanced ideas will help you be your best developer. In this blog post, we’ll detail advanced Java ideas like multithreading, concurrency, and the JVM’s inner workings. We’ll talk about how these ideas work, why they’re important for making tools that work well, and how you can use them in your own projects.
Multithreading in Java
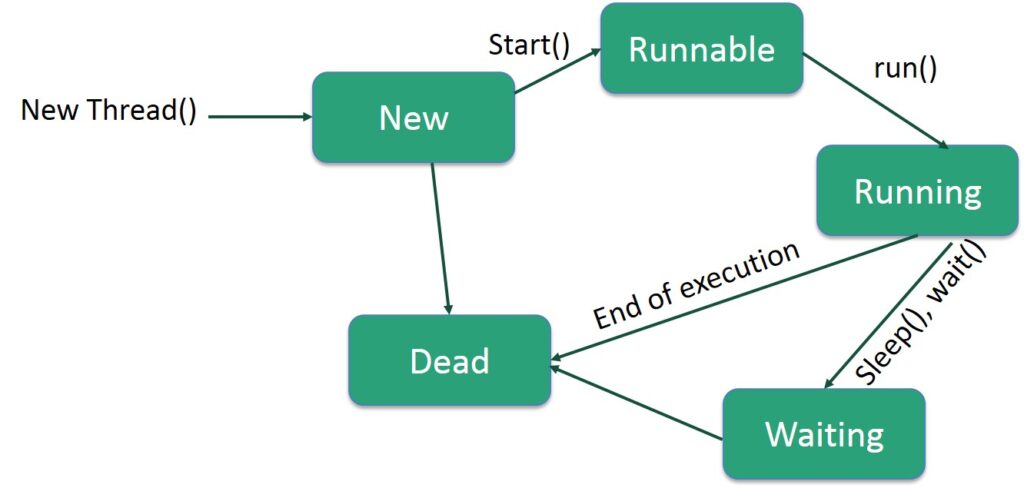
Multithreading is a technique that allows a single process to execute multiple threads concurrently. This enables better utilization of CPU resources, leading to improved performance and responsiveness in your applications.
Understanding Threads
Threads are lightweight, independent units of execution within a process, with each thread having its own stack, program counter, and local variables. They share the process’s resources, such as memory, file handles, and global variables. By utilizing threads, developers can divide complex tasks into smaller, more manageable pieces, enabling parallelism and improved application performance.
Creating Threads in Java
There are two main ways to create threads in Java:
- Extend the
Thread
class and override therun()
method. - Implement the
Runnable
interface and define therun()
method.
Here’s an example of creating a thread using both methods:
// Extending the Thread class
class MyThread extends Thread {
public void run() {
System.out.println("Thread using the Thread class");
}
}
// Implementing the Runnable interface
class MyRunnable implements Runnable {
public void run() {
System.out.println("Thread using the Runnable interface");
}
}
public class Main {
public static void main(String[] args) {
MyThread myThread = new MyThread();
myThread.start();
Thread myRunnableThread = new Thread(new MyRunnable());
myRunnableThread.start();
}
}
Thread States and Life Cycle
A Java thread can be in one of the following states:
- New: The thread has been created but has not started yet.
- Runnable: The thread is ready to run and waiting for the CPU to execute it.
- Running: The thread is currently executing.
- Blocked: The thread is waiting to acquire a lock on a resource.
- Waiting: The thread waits indefinitely for another thread to perform a particular action.
- Timed waiting: The thread is waiting for a specified period.
- Terminated: The thread has completed its execution or encountered an exception.
The life cycle of a thread consists of transitions between these states.
Thread Priorities and Scheduling
In Java, threads have priorities, which determine the order in which the scheduler executes them. Priorities range from MIN_PRIORITY
(1) to MAX_PRIORITY
(10), with the default priority being NORM_PRIORITY
(5). The scheduler uses a priority-based, preemptive scheduling algorithm, where higher priority threads are executed before lower priority threads.
Here’s an example of setting thread priorities:
MyThread myThread1 = new MyThread();
myThread1.setPriority(Thread.MIN_PRIORITY);
MyThread myThread2 = new MyThread();
myThread2.setPriority(Thread.MAX_PRIORITY);
myThread1.start();
myThread2.start();
Thread Communication
Threads can communicate with each other using shared resources, such as variables, data structures, or objects. However, care must be taken to ensure proper synchronization and avoid issues like data corruption, deadlocks, and race conditions.
Concurrency in Java
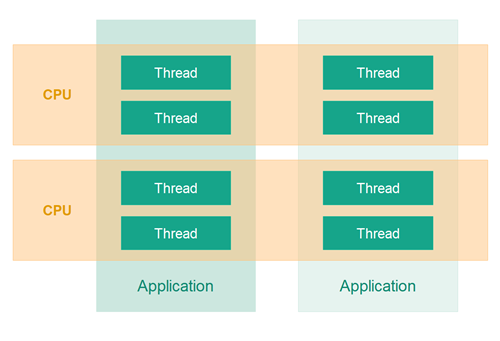
Concurrency is the simultaneous execution of multiple tasks. In Java, this is achieved through multithreading. The main challenge with concurrency is ensuring that shared resources are accessed correctly and that multiple threads don’t interfere with each other’s work. Java provides several tools and mechanisms to handle concurrent programming effectively.
Synchronization
Synchronization is used to ensure that only one thread can access a shared resource at a time. This prevents data corruption and ensures the consistency of the shared resource. In Java, synchronization is achieved using the synchronized
keyword, which can be applied to methods or blocks of code.
Here’s an example of a synchronized method:
class SharedResource {
synchronized void performTask() {
// Critical section
}
}
And here’s an example of a synchronized block:
class SharedResource {
void performTask() {
synchronized (this) {
// Critical section
}
}
}
Locks
Java provides an explicit locking mechanism through the java.util.concurrent.locks
package. The Lock
interface provides more flexibility than the synchronized
keyword, allowing you to create more complex synchronization patterns.
Here’s an example of using a ReentrantLock
:
import java.util.concurrent.locks.ReentrantLock;
class SharedResource {
private final ReentrantLock lock = new ReentrantLock();
void performTask() {
lock.lock();
try {
// Critical section
} finally {
lock.unlock();
}
}
}
Executors and Thread Pools
Java’s java.util.concurrent
package provides the Executor
framework, which simplifies the process of creating, managing, and controlling threads. Executors use thread pools to manage thread execution, allowing for better resource utilization and scalability.
Here’s an example of using an executor:
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
class Task implements Runnable {
public void run() {
// Task implementation
}
}
public class Main {
public static void main(String[] args) {
ExecutorService executor = Executors.newFixedThreadPool(4);
Task task1 = new Task();
Task task2 = new Task();
executor.submit(task1);
executor.submit(task2);
executor.shutdown();
}
}
JVM Internals
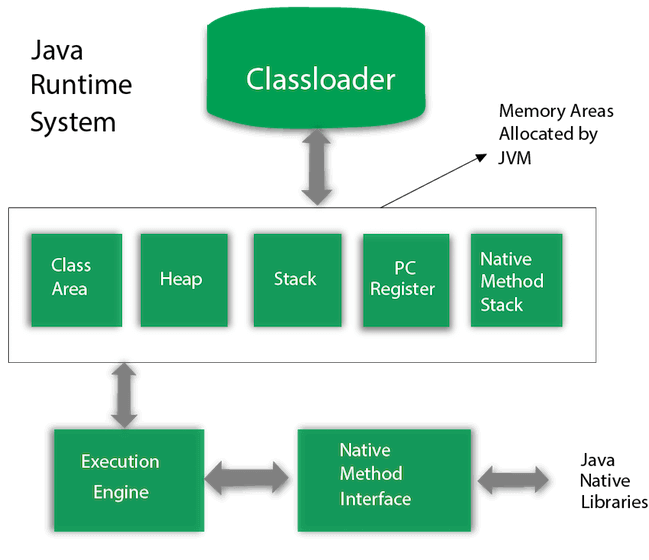
The Java Virtual Machine (JVM) is the runtime environment that executes Java bytecode. Understanding JVM internals will help you optimize your applications and manage resources more effectively.
The JVM Memory Model
The JVM memory is divided into several areas:
- Heap: This is where objects and class instances are allocated. It is further divided into the young generation, the old generation, and the permanent generation.
- Stack: Each thread in the JVM has its own stack, which stores local variables, method call information, and intermediate calculations.
- Method Area: This area stores class metadata, such as the class name, superclass, and the methods and fields of the class.
- Code Cache: The code cache is where the JVM stores compiled native code for execution.
Garbage Collection
Garbage collection is the process of automatically reclaiming memory occupied by objects that are no longer in use. The JVM performs garbage collection in multiple stages, focusing on different generations of the heap. Understanding the garbage collection process can help you manage memory more efficiently and avoid issues like memory leaks and poor performance.
Just-In-Time Compilation
The Just-In-Time (JIT) compiler is a component of the JVM that compiles bytecode into native machine code during runtime. This compilation improves the performance of the code by avoiding the overhead of interpreting bytecode at runtime.
Conclusion
By mastering advanced Java concepts like multithreading, concurrency, and JVM internals, you can significantly enhance your skills as a Java developer. Understanding these concepts will help you write efficient, high-performance applications and improve your ability to manage and optimize resources in your projects. With a strong foundation in these advanced topics, you will be better equipped to tackle complex challenges and create more robust, scalable, and responsive applications.
Key takeaways from this blog post:
- Multithreading allows for parallel execution of multiple threads within a single process, improving application performance and responsiveness.
- Concurrency in Java is achieved through multithreading and various synchronization techniques, such as the
synchronized
keyword, locks, and executors. - Understanding JVM internals, including the memory model, garbage collection, and Just-In-Time compilation, can help optimize your applications and better manage resources.
As a developer, it’s essential to keep learning and evolving. By exploring these advanced Java concepts and applying them in your projects, you’ll be better prepared to build sophisticated applications and contribute to the ever-changing world of technology.
Remember to experiment with these concepts, practice implementing them, and stay up to date with the latest developments in Java and the wider programming community. The more you learn and apply your knowledge, the more proficient you will become as a Java developer. Happy coding!