In the past few years, blockchain technology has become very popular, and it has the potential to change the way developers make and handle digital apps. Blockchain technology is a type of distributed ledger that lets multiple parties record and check events without a central authority or middleman. This technology improves security, openness, and efficiency, and software developers could use it in a number of different ways.
In this blog post, we’ll look at the basic ideas behind blockchain technology and talk about some of the ways it could be used in software development. We’ll also talk about how developers can use blockchain technology and the possible pros and cons of using it in software creation.
Fundamentals of Blockchain Technology
Before we get into how blockchain technology could be used, let’s take a look at its basic ideas. These things are:
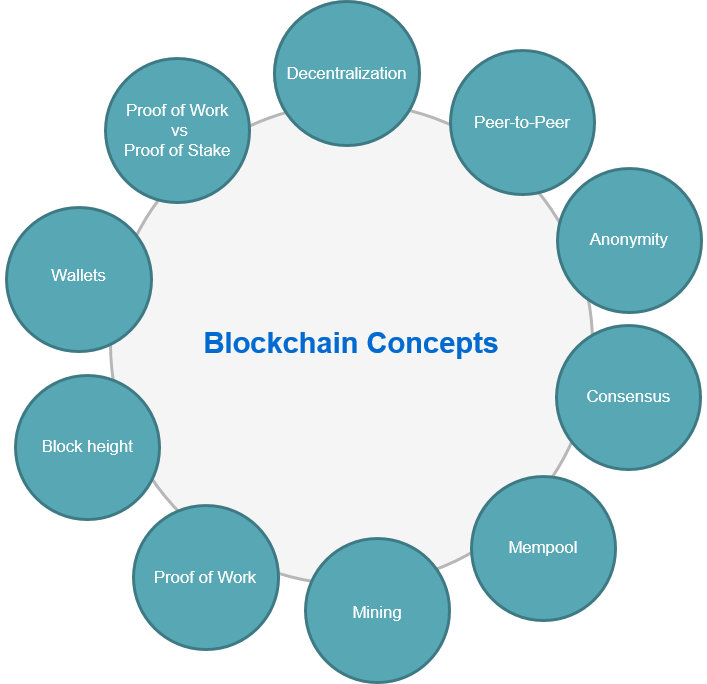
Distributed Ledger Technology: A blockchain is a type of distributed ledger that lets more than one party record and check events without a central authority or middleman. A network of nodes works together to validate events and add them to the ledger.
Consensus Algorithms: Consensus algorithms are used to make sure that all the nodes in the network agree on the state of the ledger. In blockchain technology, there are a number of consensus methods, such as Proof of Work (PoW), Proof of Stake (PoS), and Delegated Proof of Stake (DPoS).
Cryptography: Cryptography is used to protect the transactions and data kept on the blockchain. Digital signatures are used to verify transactions, and encryption is used to keep data safe.
Smart contracts: Smart contracts are contracts that run themselves and are kept on the blockchain. They make it possible for people to do business without a central authority or middleman.
Let’s look at smart contracts in more depth. Contracts that are saved on the blockchain and can run on their own are called “smart contracts.” They make it possible for people to do business without a central authority or middleman. Programming languages made just for blockchain networks, like Solidity for the Ethereum network, are used to write smart contracts.
Here’s an example of a simple Solidity smart contract:
pragma solidity ^0.8.0;
contract SimpleStorage {
uint256 public data;
function setData(uint256 _data) public {
data = _data;
}
function getData() public view returns (uint256) {
return data;
}
}
In this example, we define a simple storage contract that stores a uint256 value. The setData
function is used to set the value, and the getData
function is used to retrieve the value.
Use Cases of Blockchain Technology in Software Development
There are many ways that blockchain technology could be used in software creation. Here are some of the most popular uses:
Decentralized Applications (dApps): Decentralised applications, or dApps, are apps that are built on blockchain networks. They let people connect directly with the blockchain network, without a central authority or middleman.
Supply Chain Management: Blockchain technology can be used to improve supply chain management by making a ledger of all the events that happen in the supply chain that is both clear and safe. This can help cut down on scams and mistakes and make things run more smoothly.
Identity Verification: Blockchain technology can be used to make a system for verifying people’s identities that doesn’t depend on a single place. This system can be used to store and check information about a person’s identity, like their name, date of birth, and location. This can help stop scams and identity theft.
Digital Asset Management: Blockchain technology can be used to make a safe and clear way to manage digital assets like digital documents, tokens, and cryptocurrencies. This can help cut down on the chance of fraud and make digital asset deals run more smoothly.
Let’s take a closer look at how blockchain technology can be used in one of these use cases – supply chain management.
Supply chain management is a complicated process that involves many people, such as producers, distributors, retailers, and customers. In this process, things and services move from the person who makes them to the person who buys them. This process is often messed up by things like scams, theft, and fakes.
With blockchain technology, a ledger of all the events that happen in the supply chain can be made that is both clear and safe. Each person or business in the supply chain can record their transactions on the blockchain, and the network of nodes can check and confirm all of the transactions.
Here’s an example of a method for managing the supply chain that uses blockchain technology:
pragma solidity ^0.8.0;
contract SupplyChain {
struct Product {
uint256 productId;
string productName;
uint256 quantity;
address manufacturer;
address distributor;
address retailer;
address customer;
bool isDelivered;
bool isApprovedByDistributor;
bool isApprovedByRetailer;
}
mapping(uint256 => Product) public products;
uint256 public productId = 1;
function createProduct(string memory _productName, uint256 _quantity) public {
products[productId] = Product(productId, _productName, _quantity, msg.sender, address(0), address(0), address(0), false, false, false);
productId++;
}
function approveByDistributor(uint256 _productId) public {
require(products[_productId].isDelivered == false, "Product already delivered");
require(msg.sender == products[_productId].distributor, "Only distributor can approve");
products[_productId].isApprovedByDistributor = true;
}
function approveByRetailer(uint256 _productId) public {
require(products[_productId].isDelivered == false, "Product already delivered");
require(msg.sender == products[_productId].retailer, "Only retailer can approve");
products[_productId].isApprovedByRetailer = true;
}
function deliverProduct(uint256 _productId, address _customer) public {
require(products[_productId].isApprovedByDistributor == true && products[_productId].isApprovedByRetailer == true, "Product not approved by distributor and retailer");
require(msg.sender == products[_productId].distributor || msg.sender == products[_productId].retailer, "Only distributor or retailer can deliver");
products[_productId].isDelivered = true;
products[_productId].customer = _customer;
}
}
In this example, we define a supply chain contract that allows manufacturers to create products, distributors and retailers to approve products, and distributors or retailers to deliver products to the end customer.
The Product
struct defines the properties of a product, including the product ID, product name, quantity, manufacturer, distributor, retailer, customer, and approval status. The createProduct
function is used to create a new product, and the approveByDistributor
and approveByRetailer
functions are used to approve the product.
The deliverProduct
function is used to deliver the product to the end customer. The function checks if the product is approved by both the distributor and the retailer, and then marks the product as delivered and sets the customer address.
How Developers Can Get Involved in Blockchain Technology
There are a number of ways for developers to work with blockchain technology. Here are some of the most popular ways:
Developers can learn blockchain computer languages like Solidity and Vyper to make smart contracts and decentralised applications (dApps).
Contributing to Open-Source Blockchain Projects: Developers can help improve the functionality and speed of open-source blockchain projects like Bitcoin, Ethereum, and Hyperledger by contributing to them.
Building dApps on Blockchain Platforms: Developers can build decentralised apps (dApps) on platforms like Ethereum and Tron that use blockchain technology. These decentralised applications (dApps) can be used for different things, like managing finances, the supply chain, and games.
Here’s an example of a simple decentralised application (dApp) built on Ethereum using Solidity:
pragma solidity ^0.8.0;
contract SimpleStorage {
uint256 public value;
function setValue(uint256 _value) public {
value = _value;
}
}
In this example, we define a simple storage contract that allows users to store a value on the blockchain. The value
variable stores the value that the user sets using the setValue
function.
Developers can use tools such as Remix and Truffle to write and test their Solidity code.
Benefits and Drawbacks of Blockchain Technology
In software creation, blockchain technology has a number of possible pros and cons.
Benefits:
Better security and transparency: Blockchain technology keeps a record of all the transactions that happen on the network in a way that is both clear and safe. This makes it easy to track and confirm transactions, which makes fraud and other bad things less likely to happen.
Reduced Costs and Transaction Times: Costs and times for transactions are cut down because blockchain technology gets rid of the need for middlemen like banks and other financial companies.
Drawbacks:
Possible problems with scalability and compatibility: Blockchain technology is still in its early stages, and scaling and working with other systems are still problems. There is a chance that the current blockchain infrastructure can’t handle the number of transactions needed for large-scale apps.
Regulatory and legal challenges: Blockchain technology doesn’t follow traditional rules, which can make it hard for coders and organisations that use it to stay out of trouble.
Conclusion
In conclusion, blockchain technology could change how developers make and handle digital apps in a big way. Developers can use the benefits of blockchain technology, such as better security and transparency, to make applications that are both new and safe. But coders who use blockchain technology must also think about possible downsides, such as problems with scalability and regulations.
Developers can get started with blockchain development by learning blockchain computer languages, contributing to open-source blockchain projects, or making decentralised applications (dApps) on blockchain platforms. By doing this, developers can help shape the future of software development and drive growth in the field.