Over the past few years, the world of software creation has changed a lot. With the rise of cloud computing, microservices, and containerization, making software is harder and more complicated than ever. DevOps has become an important way for software development teams to work in a world that is changing quickly. In this blog post, we’ll talk about the best practises and tools for DevOps and how they can help your team release software faster, more efficiently, and with higher quality.
What does DevOps mean?
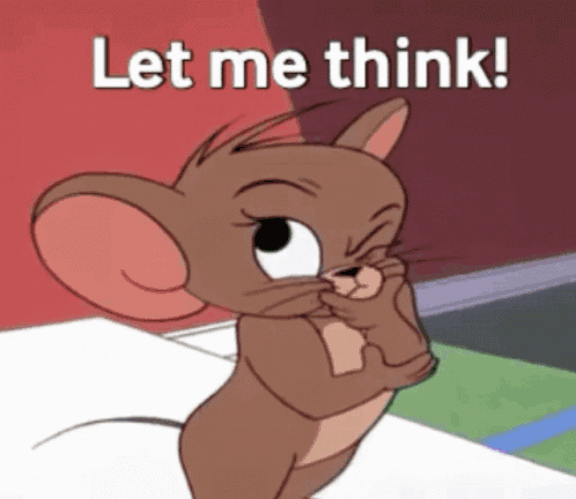
Development and Operations are both parts of DevOps. It’s a mindset, a set of practices, and a way of thinking that brings development and operations teams together to improve the software development process. DevOps’ main goal is to get software out faster and more efficiently. By breaking down walls between development and operations teams, DevOps makes it easier for people to work together and talk to each other. It also speeds up the software development process and helps teams make better software faster.
Why DevOps is important in software development
DevOps is an important part of current software development because it helps teams keep up with how quickly technology is changing. The old way of making software was slow, difficult to work with, and prone to mistakes. DevOps solves these problems by encouraging teamwork, and automation, and always getting better.
With DevOps, the development and operations teams work together to organise the whole process of making software, from writing the code to putting it into use. This system makes it easier and faster for teams to release software. DevOps also encourages continuous growth through feedback loops and metrics, which make it easier for teams to find and fix problems.
Continuous Delivery
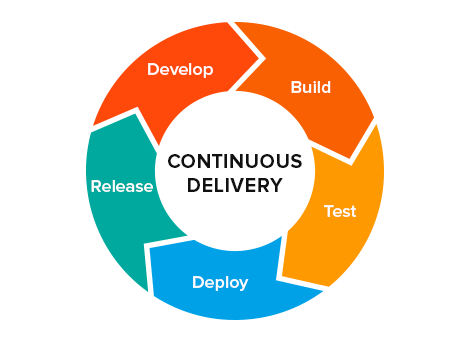
One of the most important parts of DevOps is Continuous Delivery. It’s a way of making software in which the software is built, tested, and released all by itself. Continuous Delivery lets teams put software into production quickly and often, which shortens the time it takes to get a product on the market and makes customers happier.
Best Ways to Put Continuous Delivery Into Action
For Continuous Delivery to work, both technical and behavioral changes are needed. Here are some of the best things to do when putting Continuous Delivery into place:
- Use Version Control: Use a system like Git to keep track of changes to your software.
- Automate the Build Process: To automate the build process, use build automation tools like Jenkins or Travis CI.
- Everything should be tested: To test your code, use tools like JUnit, Selenium, or Mocha that do it for you.
- Deploy Automatically: Use deployment automation tools like Ansible, Chef, or Puppet to automatically launch your software.
- Monitor Continuously: Use tracking tools like Nagios, Prometheus, or New Relic to keep an eye on your software while it’s in use.
Examples of code for Continuous Delivery
Building and Testing Code:
# build and test code using a build tool like Maven
$ mvn clean install
# run unit tests to ensure code quality
$ mvn test
Containerizing and Deploying Code:
# containerize the code using a tool like Docker
$ docker build -t myapp:v1 .
# push the container to a container registry
$ docker push myregistry/myapp:v1
# deploy the container to a Kubernetes cluster
$ kubectl apply -f deployment.yaml
Automating the Entire Pipeline:
# use a continuous integration tool like Jenkins to automate the entire pipeline
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'mvn clean install'
}
}
stage('Test') {
steps {
sh 'mvn test'
}
}
stage('Deploy') {
steps {
sh 'docker build -t myapp:v1 .'
sh 'docker push myregistry/myapp:v1'
sh 'kubectl apply -f deployment.yaml'
}
}
}
}
These are just a few examples of code that demonstrate how continuous delivery works. As you can see, continuous delivery involves automating the entire pipeline from code building and testing to containerization and deployment to monitoring and feedback. By automating these processes, you can speed up development, reduce errors, and deliver higher-quality products faster and more efficiently than ever before!
Here’s how Continuous Delivery works in the real world:
Say you are making a web program. You make some changes to the code, push them to the Git source, and tell Jenkins to run a build. Jenkins builds the program, runs the unit tests, and makes an artifact that can be deployed. Then, Jenkins sends the artifact to a staging environment where the automatic integration tests are run. Jenkins sends the program to the production environment if the tests pass.
Infrastructure-as-Code
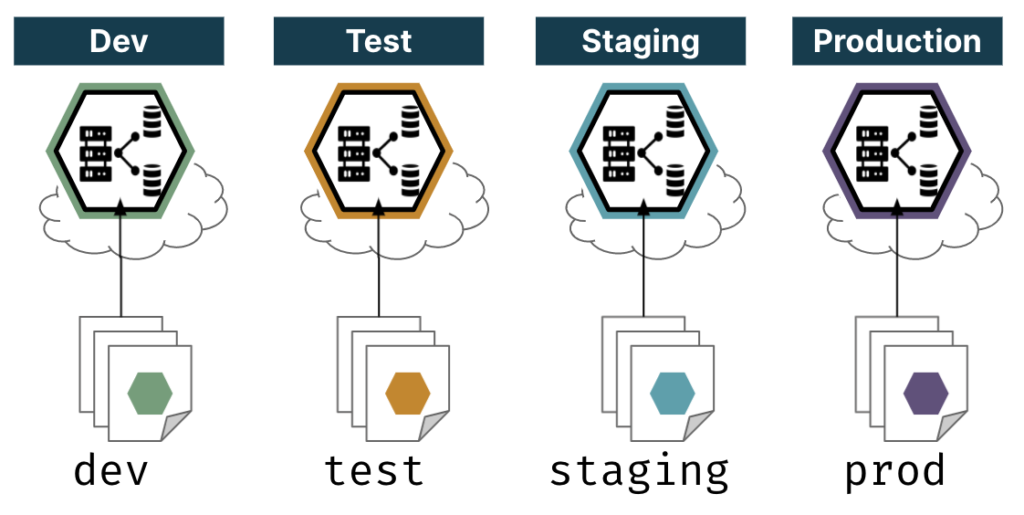
Infrastructure as Code is another important DevOps practice. It’s a method in which infrastructure is defined in code and managed using the same version control system as the application code. Infrastructure-as-Code makes it easier for teams to handle their infrastructure in a way that is more flexible and scalable, which reduces the chance of mistakes and downtime.
Definition of Infrastructure-as-Code and its Benefits
Infrastructure-as-Code is a way to handle infrastructure in which infrastructure is defined in code and managed using the same version control system as application code. Infrastructure-as-Code has the following benefits:
- Agility: Infrastructure as a Service Code lets teams handle their infrastructure the same way they manage their application code. This makes it easier to make changes and deploy them quickly.
- Scalability: With Infrastructure-as-Code, teams can control their infrastructure in a more scalable way, which makes it easier to handle larger workloads and traffic spikes.
- Consistency: Infrastructure-as-Code makes sure that infrastructure is the same in all settings. This lowers the risk of mistakes and downtime.
Tools for Implementing Infrastructure-as-Code
Infrastructure-as-Code can be put into place with a number of tools. Here are some well-known ones:
- Terraform: Terraform is an open-source tool that lets teams describe infrastructure as code and set up infrastructure in a way that is consistent and can be done over and over again.
- AWS CloudFormation: AWS CloudFormation is a managed service that lets teams describe and set up AWS infrastructure as code.
- Google Cloud Deployment Manager: Google Cloud Deployment Manager is a service that lets teams describe and deploy Google Cloud Platform resources as code. It is a managed service.
Examples of code for Infrastructure as Code
- Terraform: Terraform is a popular IaC tool that allows developers to define infrastructure as code using a high-level configuration language. The following Terraform code defines a simple infrastructure consisting of an AWS EC2 instance and an associated security group:
provider "aws" {
region = "us-west-2"
}
resource "aws_security_group" "instance" {
name_prefix = "instance-"
}
resource "aws_instance" "web" {
ami = "ami-0c55b159cbfafe1f0"
instance_type = "t2.micro"
security_groups = [aws_security_group.instance.name]
}
This code defines an EC2 instance in the us-west-2
region with the t2.micro
instance type and an associated security group.
- CloudFormation: Amazon CloudFormation is another popular IaC tool that uses JSON or YAML templates to define infrastructure as code. The following CloudFormation code defines a simple infrastructure consisting of an Amazon S3 bucket:
Resources:
MyBucket:
Type: AWS::S3::Bucket
Properties:
BucketName: my-bucket-name
This code defines an S3 bucket with the name my-bucket-name
.
- Chef: Chef is an IaC tool that uses a Ruby-based domain-specific language (DSL) to define infrastructure as code. The following Chef code defines a simple recipe to install and configure the Apache web server:
package 'httpd' do
action :install
end
service 'httpd' do
action [:enable, :start]
end
file '/var/www/html/index.html' do
content '<html><body><h1>Hello, world!</h1></body></html>'
action :create
end
This code installs the Apache web server package, starts the httpd
service, and creates a simple HTML file to serve as the web page content.
Overall, these examples demonstrate how IaC tools like Terraform, CloudFormation, and Chef can be used to define and manage infrastructure as code, making it easier to deploy and manage infrastructure at scale.
Here’s how Infrastructure as Code works in the real world:
Let’s say you run a web service on Amazon Web Services (AWS) and are in charge of it. Using Terraform, you set up your infrastructure by telling it how many instances, security groups, and load balancers you want. Then, you set up your system on AWS with Terraform. If you need to change your infrastructure, all you have to do is update your Terraform code and apply the changes. AWS will take care of the rest.
Automatic Testing and Deployment
Another important part of DevOps is automating testing and deployment. It’s a way of doing things where testing and launch are done automatically. This lets teams release software faster and better.
Explanation of Automated Testing and Deployment and Their Benefits
Automated Testing and Deployment is a method in which testing and deployment are done automatically. This makes it possible for teams to release software faster and better. Automated Testing and Deployment have many perks, such as:
- Faster release cycles: With Automated Testing and Deployment, teams can release software more quickly, which shortens the time it takes to get it on the market and makes customers happier.
- Higher quality: Automated Testing and Deployment make sure that software is fully tested. This reduces the chance of mistakes and makes the software better overall.
Best Practices for Implementing Automated Testing and Deployment
Automated Testing and Deployment need both technology and cultural changes to be put into place. Here are some tips for putting Automated Testing and Deployment into place:
- Use Test Automation Tools: To automate your testing, use tools like Selenium, JUnit, or Mocha.
- Use Continuous Integration: Automate testing and release by using a continuous integration tool like Jenkins, Travis CI, or CircleCI.
- Use Canary Releases: Use Canary Releases to slowly roll out new features to a small group of people before rolling them out to everyone.
Examples of code for Automated Testing and Deployment
- Jenkins: Jenkins is a popular open-source automation server that supports automated testing and deployment. The following Jenkins pipeline code defines a simple build, test, and deploy pipeline for a web application:
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'npm install'
}
}
stage('Test') {
steps {
sh 'npm test'
}
}
stage('Deploy') {
steps {
sh 'npm run deploy'
}
}
}
}
This code defines a pipeline with three stages: Build
, Test
, and Deploy
. In the Build
stage, the script installs the necessary dependencies using npm
. In the Test
stage, the script runs the application’s test suite using npm
. Finally, in the Deploy
stage, the script deploys the application using npm
.
- CircleCI: CircleCI is another popular continuous integration and deployment platform that supports automated testing and deployment. The following CircleCI configuration file defines a simple build, test, and deploy workflow for a Python web application:
version: 2
jobs:
build:
docker:
- image: python:3.7.4-stretch
steps:
- checkout
- run: pip install -r requirements.txt
- run: pytest
deploy:
docker:
- image: circleci/deploy-image:0.0.1
steps:
- checkout
- run: echo "Deploying application"
This code defines a workflow with two jobs: build
and deploy
. The build
job uses a Python 3.7.4 Docker image to install dependencies and run tests using pytest
. The deploy
job uses a custom Docker image to deploy the application.
- Travis CI: Travis CI is a popular continuous integration and deployment platform that also supports automated testing and deployment. The following Travis CI configuration file defines a simple build, test, and deploy workflow for a Node.js web application:
language: node_js
node_js:
- 12
script:
- npm install
- npm test
deploy:
provider: heroku
api_key: <your-heroku-api-key>
app: <your-heroku-app-name>
This code defines a workflow with two stages: script
and deploy
. The script
stage installs dependencies and runs tests using npm
. The deploy
stage deploys the application to Heroku using the Heroku API key and app name.
Overall, these examples demonstrate how continuous integration and deployment platforms like Jenkins, CircleCI, and Travis CI can be used to automate testing and deployment processes, making it easier to ensure code quality and deploy changes quickly and reliably.
Here’s a real-world example of how Automated Testing and Deployment work:
Let’s say that you are making a mobile game. You change the code and send those changes to the Git source. Jenkins builds the program, runs the unit tests, and makes an artifact that can be deployed. Then, Jenkins sends the artifact to a staging environment where the automatic integration tests are run. If the tests are successful, Jenkins deploys the application to the production system using a canary release. The new features are then slowly rolled out to a small group of users before they are rolled out to everyone.
Collaboration and Communication
Collaboration and talking to each other are very important in DevOps. It’s important to break down the barriers between the development and management teams and make sure everyone is working towards the same goal.
Importance of Collaboration and Communication in DevOps
Collaboration and communication are important in DevOps because they encourage cross-functional teamwork, which is key to the success of DevOps. Teams that work together can share their information, ideas, and feedback, which helps them make better decisions and get better results.
Best Practices for Improving Collaboration and Communication
To improve collaboration and communication in DevOps, the organization’s culture needs to change so that everyone is working towards the same goals. Here are some best practices to follow to improve collaboration and communication in DevOps:
- Foster a culture of trust: Trust is important in DevOps, and the first step is to create a safe, open space where everyone can share their thoughts and feedback without worrying about getting in trouble.
- Break down silos: Silos between teams can make it harder for them to work together and talk to each other. To get rid of silos, make cross-functional teams and encourage people to share their information.
- Use Tools for Collaboration: Use tools for collaboration like Slack, Microsoft Teams, or Zoom to help teams talk to each other.
Tools for Facilitating Collaboration and Communication
In DevOps, there are a number of tools that can help with collaboration and communication. Here are some well-known ones:
- Slack: Slack is a place where teams can talk to each other and work together in real-time.
- Microsoft Teams: Microsoft Teams is a place for working together that works with other Microsoft tools like SharePoint and Office 365.
- Zoom: Zoom is a tool for video conferencing that lets teams work together from far away.
Conclusion
In conclusion, DevOps is a set of practices and tools that help the development and operations teams work together, talk to each other, and automate tasks. Teams can release software faster and better by using best practices for DevOps, such as Continuous Delivery, Infrastructure-as-Code, Automated Testing and Deployment, and Collaboration and Communication. For these practices to work, the organization’s culture needs to change so that everyone works towards the same goals. So, if you’re not already using DevOps, it’s time to start!