Flutter app development has been an important component of the digital world in the past few years. With the need for new and easy-to-use mobile apps growing, developers are always looking for quick and easy solutions to make high-quality apps. Flutter is one of these technologies that has become very popular in the app development world.
Flutter is an open-source UI toolkit made by Google. It lets developers construct natively-compiled apps for mobile, web, and desktop from a single codebase. It uses the Dart programming language and has a lot of ready-made widgets that make it easy to construct apps that look good and work well. In this complete introduction, we’ll dive into the world of Flutter and look at all the different ways to build apps with Flutter.
Why Go with Flutter?
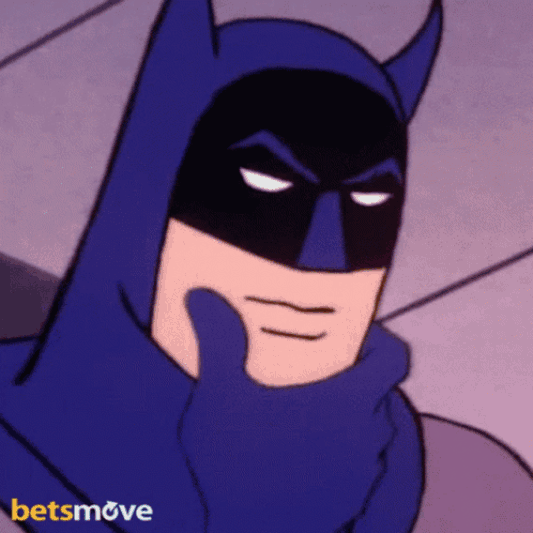
Before we go into the basics of how to grasp Flutter, let’s look at why it has become so popular among app developers.
- Cross-Platform Development: One of the best things about Flutter is that you can use a single codebase to make apps for many platforms. This implies that you only have to create code once, and it will work on Android, iOS, the web, and your desktop. This makes development faster and easier.
- Fast and Native: Flutter directly compiles Flutter apps into native code, enabling them to run quickly with smooth animations. Additionally, it includes a plethora of pre-built widgets that adhere to the Material Design and Cupertino design guidelines. This gives your app a native look and feels on both Android and iOS.
- Hot Reload: Hot Reload is a feature of Flutter that lets developers see changes to the code in real-time without having to restart the app. This makes the development process very productive and iterative, so developers can quickly try out new ideas and make changes.
- Customizable UI: Developers can change a vast selection of UI components in Flutter to create distinctive and visually appealing interfaces. Flutter also offers a flexible layout system adapted to various screen sizes and orientations. This makes it easier to make apps that are responsive.
- Strong Community Support: Flutter has a huge and active community of developers, which means you can discover a lot of tools, tutorials, and plugins to help you build apps. Since the community is always changing and developing, Flutter is a good choice for making apps.
Now that we know why Flutter is a good choice let’s look at the most important ideas and features of Flutter app development.
Key Ideas About Flutter
- Widgets: Flutter makes apps using widgets. Flutter has two types of widgets: stateless widgets, which cannot be changed after creation, and stateful widgets, which can be changed and updated in real time while the program is running. Widgets are the building blocks of the app’s user interface and can be combined to create complex UI layouts.
- Material Design and Cupertino: Flutter builds Android and iOS apps by following the Material Design and Cupertino design rules. Material Design is a set of rules for making UI that is visually appealing, responsive, and consistent. Cupertino is a set of UI components that seem like they belong on iOS. Flutter comes with a lot of Material Design and Cupertino widgets that make it easy to develop stunning apps that appear like they were made for the device.
- Layouts: Flutter has a strong and versatile layout framework that lets developers make UI layouts that are responsive and adaptable. Container, Row, Column, Stack, and Expanded are some of the layout widgets that are used most often in Flutter. These widgets give you different ways to put things on the screen, like text, photos, and buttons.
- State Management: Managing an app’s state is an important part of making apps. State management in Flutter can be done in a number of ways, such as with StatefulWidget, InheritedWidget, Provider, and Redux. These methods help manage the app’s state and make sure that the UI always matches the data.
- Navigation: Flutter has a built-in navigation system that developers may use to control how their app’s navigation works. Moving between different displays or pages in an app is called navigation. Flutter has a widget called Navigator that allows you to manage the navigation stack and go from one screen to the next. It also has route management, which lets developers set up routes and govern how users move from one screen to another in a logical and organised way.
- Animation: Flutter features a robust animation framework that makes it easy for app developers to make animations that are smooth and interactive. It gives you a lot of animation widgets and classes, such AnimatedContainer, AnimatedOpacity, and Tween, that you can use to make animations that look good. The animation framework in Flutter makes it easy to add fun and interesting animations to your app, which improves the user experience as a whole.
- Plugins: Flutter has a huge ecosystem of plugins that add to its capabilities and give access to other APIs and device features. The community takes care of Flutter plugins, which have a wide range of functions, such as accessing device sensors, calling APIs, handling authentication, and more. These plugins save time and effort on development by having ready-made solutions for common tasks and integrations with services from other companies.
Mastering Flutter: A Step-by-Step Guide
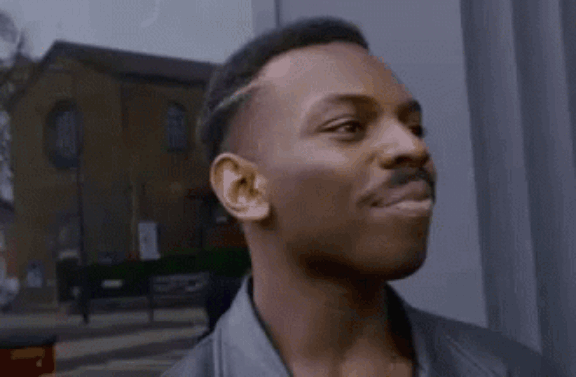
Now that we’ve talked about Flutter’s main ideas let’s look at a step-by-step tutorial on making apps using Flutter.
Step 1: Setting up Flutter
Set up your development environment to get started with Flutter. Here’s how to do it:
- Install Flutter: The official Flutter website lets you download and install Flutter. Flutter has installation directions for Windows, macOS, and Linux, among other platforms.
- Set up an IDE: Flutter works with many IDEs (Integrated Development Environments), such as Visual Studio Code, Android Studio, and IntelliJ IDEA. You can choose the IDE you are most familiar with and install the Flutter and Dart plugins to start making Flutter apps.
Step 2: Understanding Flutter’s Widget Tree
As we’ve just talked about, a Flutter app is made up of widgets. To make a Flutter app, you need to know how the widget tree works. Here are the most important parts of the widget tree in Flutter:
- Widget Hierarchy: In Flutter, widgets are put together in a way that looks like a tree. This is termed the widget tree. The widget at the top of the tree is called the root widget, and all of the widgets below it are called its offspring. Widgets can have one or more children, which makes a framework with levels.
- Composing Widgets: You can put widgets together to make complicated user interface layouts. Flutter comes with a lot of pre-made widgets, such Text, Image, Button, Container, and more, that may be put together to make a bespoke layout for the user interface. You can also make your own widgets by adding to existing ones or putting together more than one widget.
- Widget State: In Flutter, widgets can either have no state or have a state. Once they are made, stateless widgets can’t be changed, but stateful widgets can. Managing the state of widgets is an important part of making Flutter apps.
Step 3: Making a user interface with widgets
Once you know how the widget tree works in Flutter, the next step is to use widgets to construct the user interface of your app. Here are the most important steps for using widgets to develop UI:
- Choose the Right Widgets: Flutter has a large number of widgets that are already made and match the Material Design and Cupertino design rules. Choose the right widgets for your app’s user interface (UI) elements, like text, photos, buttons, input fields, and more. With their attributes and parameters, you can also change how the widgets seem.
- Set Up a Hierarchy for Widgets: As we’ve already said, widgets in Flutter are set up in a tree-like structure. Set up your app’s user interface layout so that the root widget is at the top and its children’s widgets are below it. This helps make a more organised and structured UI layout.
- Use Layout Widgets: Flutter has a number of layout widgets that can help you arrange the UI elements in a way that looks good and works well. Complex UI layouts can be made with layout widgets like Container, Column, Row, Stack, and others. You can change the layout of these layout widgets to fit the needs of your app by using their settings and parameters.
- Handle User Input: User input is a key part of every project, and Flutter has widgets to handle text input, button presses, gestures, and more. You can handle user input in your app with widgets like TextField, ElevatedButton, InkWell, GestureDetector, and more.
Step 4: Managing App State
Managing your app’s state is a key part of making dynamic, interactive apps. Flutter has many ways to control the state of widgets and the state of the app as a whole. Here are some important ideas about how to handle app state in Flutter:
- Stateful Widgets: In Flutter, stateful widgets are widgets that can alter while the app is running. These widgets keep their state, which can be changed and shown in the user interface (UI). To make a stateful widget in Flutter, you extend the StatefulWidget class and implement the createState() method to make a mutable state object.
- State Management approaches: Flutter includes numerous approaches for managing app state, including as setState(), Provider, BLoC (Business Logic Component), Redux, and more. You may control the state of widgets and the state of the app as a whole in a logical and organised way with these methods. Choose the right state management method based on how complicated your app is and what it needs to do.
Reactive programming is a way of managing app state that is more responsive and efficient. Flutter comes with a framework for reactive programming called RxDart. This lets you use reactive programming patterns like Streams and Observables in your app. RxDart can be utilised with other state management approaches to make apps that are both reliable and scalable.
Step 5: Use the navigation tools
The ability to move between multiple screens or pages in an app is a crucial part of app development. Flutter comes with a built-in navigation mechanism that makes it easy to control how your app navigates. Here are the main things you need to do to deal with navigation in Flutter:
Routes are the screens or pages that can be navigated to your app. Use the Navigator widget, which lets you add and remove routes from the navigation stack, to set up routes in your project. You may give route names, which makes it easy to administer your app and move between different panels.
- Handle Navigation Events: Flutter includes callbacks and events for handling navigation events, such as when a route is pushed, popped, or replaced. Use these callbacks and events to handle navigation events in your app, like changing the UI, managing the app’s state, and more.
- Navigation Transitions: Flutter has built-in transitions like FadeTransition, SlideTransition, and more for moving between screens. Using Flutter’s animation framework, you can make changes to these transitions or make your own. Navigation transitions give a visually pleasing touch to your program, boosting the user experience.
Step 6: Adding Animations
Animations are a terrific technique to make your app more interactive and interesting to look at. Flutter features a strong animation framework that lets you make animations that are seamless and interesting. Here are the steps you need to take in order to add animations to your Flutter app:
- Choose Animation Widgets: Flutter has many animation widgets, such as AnimatedContainer, AnimatedOpacity, AnimatedPositioned, and more, that may be used to animate different UI elements in your app. Choose the right animation widgets depending on what kind of animation you want to make. Animation controllers are used to make your app’s animations work the way you want them to. They set the animation’s length, curvature, and other features. The Flutter AnimationController class can be used to make animation controllers.
- Create Animation Tweens: Animation tweens set the values of the animation’s beginning and end. The Tween class that comes with Flutter can be used to make animated tweens. Tween lets you set the animation’s settings in a smooth and gradual way.
- Attach Animation Controllers and Tweens to Animation Widgets: Once you’ve set up your animation controllers and tweens, you can attach them to animation widgets using the animation property that these widgets offer. This connects the animations to the UI elements and uses the values set in the animation controllers and tweens to control the animation.
- Handle Animation Events: Flutter has callbacks and events for handling animation events, like when an animation starts, ends, or changes its value. You may utilise these callbacks and events to update the user interface, change the state of the app, and make animations that users can interact with.
Step 7: Testing and Debugging
Testing and debugging are key steps in the process of making an app to make sure it works as planned and has no bugs or other problems. Flutter has tools and libraries built in that you can use to test and fix your project. Here are some important ideas to keep in mind when testing and fixing bugs with Flutter:
Unit testing is the practice of testing small pieces of code, like functions or methods, to make sure they perform as expected. Flutter has a built-in testing framework called Flutter Test that lets you develop and run unit tests for your app’s code. By writing unit tests, problems in the code can be found and fixed early on in the development process.
Widget Testing is the process of testing each widget on its own to make sure it looks right and responds to user inputs the way it should. Flutter has a testing framework called Flutter Widget Test that you may use to write and execute tests for your app’s user interface. Writing widget tests help you find and correct problems with your app’s UI layout and how things work.
- Integration Testing: This is the process of testing how multiple aspects of your program, like different screens, state management, navigation, and more, work together. Flutter comes with a testing framework called Flutter Driver that lets you write and run integration tests for how your app’s parts function together. Integration tests help find and address problems with how the software flows and interacts as a whole.
- Debugging: Flutter has a robust debugging tool called Flutter DevTools that lets you look at your app’s code, state, user interface (UI), and performance and find bugs in them. You can use Flutter DevTools to find problems in the code and behavior of your app and solve them, such as null pointer exceptions, wrong state values, and more.
Step 8: Getting the best results
Performance optimisation is a key part of making programs that run smoothly and respond quickly. Flutter gives you a lot of tips and tricks for making your app run as well as possible. Here are some important ideas for getting the most out of Flutter:
- Rebuilding Widgets: Flutter uses a reactive programming style, which means that the user interface is rebuilt anytime the app state changes. But rebuilding widgets that don’t need to be rebuilt will slow down your app. You can speed up the process of rebuilding widgets by leveraging things like const widgets, the shouldRebuild property of widgets, and more.
- Layout Optimization: Flutter has a number of layout widgets that help organise the user interface elements in a way that looks good and works well. But using layout widgets in the wrong way can hurt the way your program works. You may improve layout efficiency by using the right layout widgets for your user interface design, avoiding nested layouts, employing constraints and aspect ratios, and other methods.
- Image optimisation: photos are an important aspect of many apps, however, huge photos might slow your app down. You can improve the efficiency of photographs by doing things like scaling them to the right size, compressing them, utilising the right image format, and more.
- State Management Optimization: Managing states well is a key part of making your program run as well as possible. Flutter offers different ways to handle state, like Provider, BLoC, Redux, and more. You can improve state management by doing things like limiting the number of changes that don’t need to be made, updating only certain states, loading data slowly, and more.
- Performance Profiling: Flutter has built-in performance profiling tools like Flutter DevTools that let you look at your app’s performance and find slow spots. You can use tools for performance profiling to find parts of your program that could be faster and make the changes you need.
Step 9: Making it work in different places
Localization and internationalisation are important parts of making an app, especially if you want to reach people from different countries and languages. Flutter has built-in support for localization and internationalization, so you can make apps that can be used by people from all over the world. Here are some important ideas about localization and going global in Flutter:
- Localizing Text: Flutter lets you utilise the built-in Dart intl package to localise text in your app. This package helps you format dates, numbers, currencies, and other things dependent on the user’s locale. You can create localised text resources in separate localization files and load the right resource based on the user’s locale. Images may be localised in your app using Flutter by giving distinct images with varied resolutions for different locales. You can declare localised image resources in separate localization files and load the right picture based on the user’s locale.
- Right-to-Left (RTL) Support: Flutter has built-in support for languages that are read from right to left, so you can make apps that people who read from right to left may utilise. You can add RTL support to your app by leveraging Flutter’s built-in RTL support or by making layout and UI changes yourself to support RTL.
- Internationalizing App Configuration: Flutter lets you change things like date formats, number formats, and more so that they work in different places. You can create internationalised app configuration in separate localization files and load the right configuration based on the user’s locale.
- Testing Localization: Flutter has built-in tools for testing your app’s localization, so you can make sure it shows the right localised content based on the user’s locale. You may use the built-in testing tools to make sure that your app is localised correctly and shows the right content for different places.
Step 10: Publish and get the word out
After you’ve made and tested your app, the last thing to do is publish and send it to users. Flutter gives you different ways to publish and distribute your app on different platforms, such the App Store, the Google Play Store, and more. Here are some important ideas to keep in mind as you publish and spread your Flutter app:
- Publishing to the App Store and Google Play Store: Flutter lets you publish your app to the App Store and Google Play Store in the same way that iOS and Android apps usually do. You can publish your app to Apple or Google’s app stores by following the instructions and requirements they give you.
- App Signing and Release Management: Flutter gives you tools and instructions for signing your app and managing its releases. This lets you be sure that your app is safe and secure while it is being published. You can create signed APKs or app bundles for distribution with Flutter commands like flutter build, flutter build apk, flutter build appbundle, and more.
- App Distribution Platforms: Besides the App Store and the Google Play Store, you can also use Firebase App Distribution, TestFlight, HockeyApp, and other platforms to get your Flutter app out there. These systems let you give your app to individual testers or groups so they may try it out and give you feedback before it comes out to the public.
- Software Store Optimization (ASO): Just like with any other software, your Flutter app needs to be optimised for the app store so that potential consumers can find it and see it. You may boost your app’s rating in the app store search results and make it more likely to be downloaded by tweaking its metadata, keywords, screenshots, and more.
- Updates and maintenance: Once you’ve published your Flutter app, it’s crucial to update and maintain it often to solve bugs, add new features, and make it run better. Flutter gives you tools and instructions for updating and maintaining your app, such as hot-reloading, hot-restarting, and more. These let you make changes to your app without having to do a full update.
Conclusion
Flutter is a robust and flexible app development framework that lets you design apps that function on more than one platform from a single codebase. In this detailed book, we’ve gone over the most important ideas and steps you need to know to grasp Flutter for app development. You now have a good understanding of the full app development lifecycle with Flutter, from setting up the development environment to developing UIs, handling state management, optimising performance, localising and internationalising apps, publishing and distributing apps, and more.
By following best practices, taking advantage of the many widgets and libraries that Flutter provides, and always learning and developing your abilities, you can become a skilled Flutter developer and make high-quality apps for several platforms. Always check the official Flutter documentation and community resources for the latest developments and best practises in Flutter development. Have fun writing code and making great apps with Flutter!