As we tread into the realm of systems programming, safety, and concurrency become fundamental requirements. Traditionally, this meant choosing either performance or safety. Rust, however, promises both. With its unique memory management and concurrency approach, Rust is quickly gaining traction as a powerful, reliable, and efficient programming language. This blog post will guide you through the key features of Rust, how it ensures memory safety? and how it enables fearless concurrency.
What is Rust?
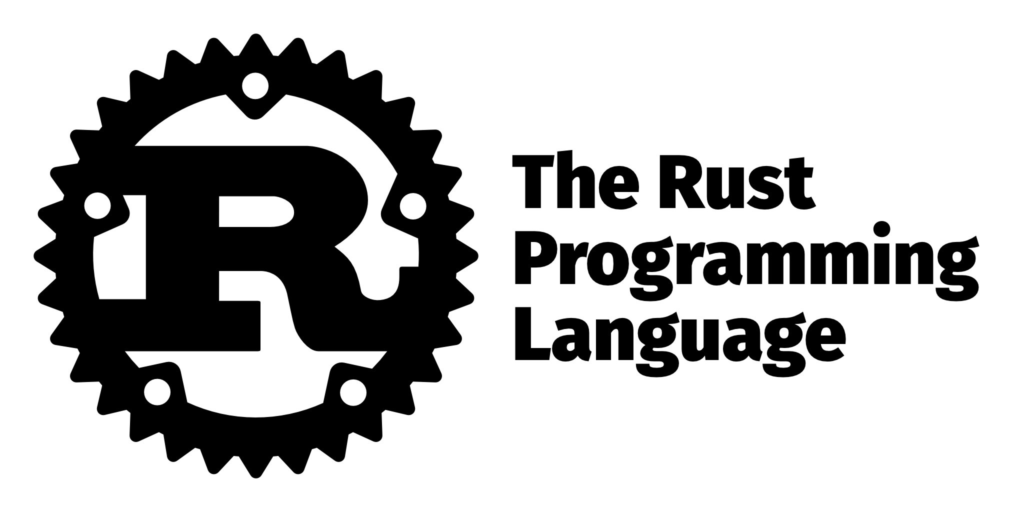
Rust is a multi-paradigm, statically-typed, compiled language conceived for systems programming. Graydon Hoare at Mozilla Research, with contributions from the open-source community and Mozilla, designed Rust. The fundamental philosophy behind Rust is to ensure memory safety without sacrificing performance, which it achieves through a series of rigorous checks at compile time, negating the need for a garbage collector. Rust is syntactically similar to C++, but its design allows developers to write safer and faster code.
Why Rust?
The core strength of Rust lies in its focus on speed and memory safety. Rust has a unique way of ensuring memory safety – it eliminates null and dangling pointers, two notorious sources of bugs in C++ programs. Dangling pointers or wild pointers in computer programming are pointers that do not point to a valid object of the appropriate type. In Rust, however, these errors are caught during compile time, resulting in safer code.
Rust also provides safe ways to work with memory and concurrency, allowing developers to write high-performance code with fewer risks. Its syntax is friendly to developers familiar with C-based languages, making it a strong candidate for those seeking a language that offers safety without compromising on performance.
Understanding Ownership in Rust
Ownership is a key feature in Rust that separates it from many other languages. It significantly affects how Rust handles memory management without a garbage collector.
What is Ownership?
The concept of ownership in Rust is based on three key rules: each value in Rust has a variable that’s its owner, there can only be one owner at a time, when the owner goes out of scope, the value will be dropped. Rust’s ownership model is a powerful tool that helps prevent bugs and ensure more predictable and safer code.
For instance, consider this example:
fn main() {
let s = "hello"; // s is in scope here and 'owns' the value "hello"
} // s goes out of scope here, and Rust cleans up the memory
In this code snippet, the string literal “hello” is owned by the variable s
. When s
goes out of scope at the end of the main
function, Rust automatically frees the memory that was used to store “hello”. This mechanism prevents memory leaks, as there are no stray references that the garbage collector must track down later.
Borrowing and References
Rust allows the borrowing of values through the use of references, which allows you to use values without taking ownership of them. This feature is particularly beneficial when you want to access data without taking ownership or making a duplicate copy of the data.
Example:
fn main() {
let s1 = String::from("hello");
let len = calculate_length(&s1);
println!("The length of '{}' is {}.", s1, len);
}
fn calculate_length(s: &String) -> usize {
s.len()
} // s goes out of scope here, but it does not have ownership so nothing happens
In this example, s1
is passed by reference (not by value) to the function calculate_length
. This function takes a reference to a string and returns its length. Since we are borrowing s1
and not taking ownership of it, s1
remains valid after the call to calculate_length
. We can use it in the next line to print it to the console. This powerful feature of Rust ensures memory safety and efficient usage of resources.
Fearless Concurrency with Rust
Concurrency in Rust is one of the standout features of the language. While concurrency in other languages can often lead to complex and hard-to-debug issues, Rust’s memory safety guarantees make it far easier to write concurrent code.
Concurrency with Threads
The basic unit of execution in Rust is a thread. By default, Rust gives you fine-grained control over when and how new threads are created. Each thread is isolated and has its own stack and local state. This isolation is key to Rust’s ability to prevent data races at compile time.
Consider this example:
use std::thread;
use std::time::Duration;
fn main() {
let handle = thread::spawn(|| {
for i in 1..10 {
println!("hi number {} from the spawned thread!", i);
thread::sleep(Duration::from_millis(1));
}
});
for i in 1..5 {
println!("hi number {} from the main thread!", i);
thread::sleep(Duration::from_millis(1));
}
handle.join().unwrap();
}
This code creates a new thread that executes a closure, printing a statement 10 times with a pause in between each one. In the meantime, the main thread also prints a similar statement 5 times. This concurrent execution can lead to various outputs, depending on how the operating system schedules the threads.
Message Passing
A central idea of concurrent programming is the concept of message passing, where threads communicate by sending each other messages. This strategy avoids shared state and the resulting possibility of data races. Rust provides first-class support for message passing through channels.
Let’s look at how channels work in Rust:
use std::sync::mpsc;
use std::thread;
fn main() {
let (tx, rx) = mpsc::channel();
thread::spawn(move || {
let val = String::from("hi");
tx.send(val).unwrap();
});
let received = rx.recv().unwrap();
println!("Got: {}", received);
}
Here, we first create a channel using mpsc::channel
. This method returns a tuple of a transmitter and a receiver. The transmitter tx
is then moved into a new thread where it’s used to send a string message. In the main thread, we call recv
on the receiver rx to wait for a message. When the message arrives, it’s printed out. This example illustrates how you can safely use message-passing concurrency to send data from one thread to another.
Memory Safety and Zero-Cost Abstractions
Rust’s foremost promise is its emphasis on memory safety without sacrificing performance. This language allows you to manipulate hardware as a systems programming language directly, yet it also ensures memory safety and provides zero-cost abstractions.
Memory Safety
Rust’s design aims to eliminate common programming errors like null pointer dereferencing, double free memory errors, and data races. It achieves this through a series of checks and balances at compile time and does not require a garbage collector, offering deterministic performance.
One of the most common errors in other programming languages is the use of uninitialized or null pointers. In Rust, however, this class of bugs is largely avoided because Rust enforces the initialization of variables and prevents null values through the use of its Option<T>
type.
Consider the following code:
fn main() {
let mut data: Option<String> = None;
println!("Data: {:?}", data);
data = Some(String::from("Hello, world!"));
println!("Data: {:?}", data);
}
In this example, we initially set data
to None
, which is Rust’s way of expressing the absence of a value (a concept similar to null in other languages). If you try to use data
while it’s still None
, Rust’s type system will prevent you from doing so, thus preventing null pointer bugs.
Zero-Cost Abstractions
One of Rust’s main design principles is “zero-cost abstractions.” This means that you can use high-level abstractions without incurring runtime overhead. Rust achieves this by doing more work at compile time, which might make compilation slower but results in faster executables.
Consider this example:
fn main() {
let mut numbers: Vec<i32> = Vec::new();
for i in 1..1000000 {
numbers.push(i);
}
let large_sum: i32 = numbers.iter().sum();
println!("The sum of numbers from 1 to 1,000,000 is {}", large_sum);
}
In this example, we’re using a Vec<i32> to store one million numbers and then using an iterator and the sum method to calculate the sum of all numbers. This code is written in a high-level style, but it’s executed with the efficiency of low-level code. The complex logic is abstracted away by Rust’s zero-cost abstractions, resulting in code that’s easier to write and understand but doesn’t sacrifice performance.
Conclusion
Rust is a powerful, modern programming language that has made waves in the world of systems programming. Its focus on memory safety, zero-cost abstractions, and concurrency enables developers to write high-performance, concurrent code that is free from common bugs.
Rust’s ownership model and its unique approach to managing memory and concurrency make it a compelling choice for system-level programming. Its syntax is familiar and friendly to those accustomed to C-based languages, while its comprehensive standard library and package manager provide convenience and ease of use.
In the years to come, Rust is poised to shape the future of system programming, becoming the go-to language for creating reliable, efficient, and secure systems. Whether you’re an experienced system programmer looking to level up your coding game or a newcomer to system programming searching for a safe and powerful language to learn, Rust has much to offer. So why not give it a try and experience the power of Rust for yourself? Happy Rust-ing!