In the ever-evolving landscape of technology, system programming stands as a foundational pillar, driving the core functionalities of our computing devices. It is the art of crafting software that manages and maximizes hardware performance, ensuring efficient, low-level operations. This domain has traditionally been dominated by languages like C and C++, known for their power and control over system resources.
Enter Rust, a modern programming language that promises the same level of control without compromising on safety. Born out of the need for memory safety and concurrency management, Rust has quickly risen to prominence, offering a compelling alternative in system programming. Its unique approach to memory management, zero-cost abstractions, and fearless concurrency has caught the attention of developers and companies alike.
The emergence of Rust in web development is a testament to its versatility and robustness. With the advent of WebAssembly, Rust has found a new arena where its performance and safety features shine. It allows developers to write fast, secure code for the web, pushing the boundaries of what’s possible in browser-based applications.
As we delve deeper into the capabilities of Rust, we’ll explore how it’s changing the game in web development, making applications safer, faster, and more reliable.
Why Rust?
Rust is a language that’s capturing the attention of developers worldwide, and for good reasons. It’s designed to provide memory safety and high performance, all while fostering a vibrant community and ecosystem. Here’s why Rust is becoming a preferred choice for many programmers:
Memory Safety Features
One of the most significant advantages of Rust is its commitment to memory safety. The language’s ownership model, along with its borrowing and lifetimes concepts, ensures that memory is managed safely and efficiently. This design eliminates common issues such as null pointer dereferencing and data races, which are prevalent in other system programming languages. By enforcing these rules at compile time, Rust guarantees that any code that could lead to such errors is addressed before runtime.
Performance Akin to C and C++
Rust doesn’t just prioritize safety; it also delivers performance that can rival, and sometimes surpass, that of C and C++. It provides developers with the tools to control low-level system details, enabling the creation of highly optimized code. This performance is achieved without sacrificing safety, making Rust a compelling option for projects where efficiency is critical.
Growing Community and Ecosystem
The Rust community is rapidly expanding, with an increasing number of developers contributing to its ecosystem. This growth is supported by a wealth of libraries and tools that make developing in Rust more accessible and enjoyable. The language’s focus on collaboration and inclusivity has helped cultivate a supportive environment where both new and experienced programmers can thrive.
Rust in Web Development
Rust is making significant inroads into web development, a field traditionally dominated by languages like JavaScript, Python, and Ruby. Here’s how Rust is being used in web development and how it stands out when compared to these traditional languages:
How Rust Can Be Used in Web Development
Rust is being leveraged to build robust backend services as well as performant frontend applications. With its compatibility with WebAssembly, Rust code can be executed in the web browser, offering near-native performance. Frameworks such as Rocket for the backend and Yew for the frontend enable developers to write full-stack applications entirely in Rust. This opens up new possibilities for web development, where the performance and safety of Rust can be utilized to create more secure and efficient web applications.
Comparison with Traditional Web Development Languages
Compared to traditional web development languages, Rust offers several advantages:
- Performance: Rust’s performance is comparable to that of C and C++, which is beneficial for compute-intensive operations on the web.
- Memory Safety: Rust’s strict ownership and borrowing rules ensure memory safety, reducing the risk of security vulnerabilities that can arise from improper memory management.
- Concurrency: Rust’s safe concurrency model and async/await features are well-suited for handling multiple web requests efficiently, which is essential for web services.
- Modern Language Features: Rust includes modern language features like pattern matching and closures, which can lead to more expressive and maintainable code.
However, Rust also has its challenges:
- Learning Curve: Rust’s unique features such as ownership and lifetimes can present a steeper learning curve for new developers.
- Ecosystem: While Rust’s web development ecosystem is growing, it is not as mature as that of JavaScript, which has a vast array of libraries and frameworks.
Rust is a promising option for web development, especially for projects where performance and safety are critical. Its growing ecosystem and modern language features make it an increasingly attractive choice for developers looking to build full-stack applications.
Getting Started with Rust
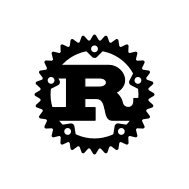
Setting up a development environment for Rust is straightforward. Here’s a step-by-step guide to get you started:
- Install Rust: The first step is to install Rust using
rustup
, which is the Rust installer and version management tool. You can download it from the official Rust website. - Choose an IDE: For writing Rust code, you can use various IDEs or text editors. Visual Studio Code is a popular choice with great Rust support through extensions like
rust-analyzer
. - Configure Rust Toolchain: Rust has different release channels (stable, beta, and nightly). For web development, you might need the nightly toolchain for certain features.
- Install C++ Build Tools: On Windows, you’ll need to install the Microsoft C++ Build Tools, which are required by some dependencies in Rust projects.
- Hello World Program: Start with a simple “Hello, World!” program to ensure your setup is correct. Create a new project using
cargo new hello_world
, then build and run it withcargo run
. - Explore Cargo: Learn how to use Cargo, Rust’s build tool and package manager. It helps in managing dependencies, building projects, and running tests.
Basic Rust Programs for Web Development
Once your environment is set up, you can start exploring Rust for web development:
- Create a Simple Web Server: Use frameworks like
Rocket
orActix
to create a basic web server that responds to HTTP requests. - Build a REST API: Implement a RESTful API by defining routes and handlers that process different HTTP methods and return JSON responses.
- Work with Databases: Learn to integrate databases using ORMs like
Diesel
or asynchronous libraries likesqlx
for data persistence. - Asynchronous Programming: Rust’s
async/await
syntax allows you to write non-blocking code, which is essential for scalable web services. - WebAssembly: Explore how Rust can be used with WebAssembly to run high-performance code in the browser.
By following these steps, you’ll be well on your way to developing web applications with Rust. Remember to refer to the official Rust documentation and community resources for more detailed guides and best practices.
Integrating Rust with Other Technologies
Integrating Rust with other technologies, particularly JavaScript and WebAssembly, is a powerful way to enhance web applications with Rust’s performance and safety features. Here’s a high-level overview of how these integrations can be achieved:
Interfacing Rust with JavaScript and WebAssembly
- Rust can be compiled into WebAssembly (Wasm), allowing it to run in the web browser alongside JavaScript.
- The
wasm-bindgen
tool facilitates communication between Wasm modules and JavaScript, enabling Rust functions to be called from JavaScript and vice versa. - This integration allows developers to write performance-critical sections of their web applications in Rust while leveraging the extensive ecosystem of JavaScript for the rest of the application.
Building Backend Services with Rust
- Rust is well-suited for backend development due to its performance and safety guarantees.
- Frameworks like Actix and Rocket provide the necessary tools to create robust API services with Rust.
- Rust’s async/await syntax and powerful concurrency model make it ideal for handling high-throughput and low-latency backend services.
- Integrating Rust with databases and other backend technologies is facilitated by a growing number of libraries and tools in the Rust ecosystem.
By leveraging Rust’s strengths in both frontend and backend development, you can create highly efficient and secure web applications. The combination of Rust with JavaScript and WebAssembly on the frontend, and its use in backend services, provides a full-stack development experience that can meet the demands of modern web applications.
Challenges and Considerations
When adopting Rust for web development, there are several challenges and considerations to keep in mind:
Learning Curve
- Rust has a reputation for having a steep learning curve. This is often attributed to its unique features such as ownership, borrowing, and lifetimes, which are different from those in more commonly used languages.
- The language’s strict compiler enforces these rules to ensure memory safety, which can be challenging for newcomers to grasp.
- However, once these concepts are understood, they provide a powerful toolset for writing safe and efficient code.
Integration with Existing Systems
- Integrating Rust with existing systems, especially those written in C or C++, can be complex but is feasible due to Rust’s interoperability with C.
- Strategies for incorporating Rust into existing projects include creating Rust libraries that can be called from C/C++ code, or vice versa.
- Assessing the trade-offs and benefits of using Rust alongside other languages is crucial. While Rust offers performance and safety benefits, it requires careful planning to integrate with the existing codebase without disrupting the current system’s functionality.
By considering these challenges and planning accordingly, developers can successfully leverage Rust’s capabilities in their web development projects, leading to more reliable and performant applications.
Conclusion
As we look to the future, Rust’s role in web development seems poised for growth. Its unparalleled focus on safety, speed, and concurrency positions it as an ideal candidate for building the next generation of web applications. The language’s ecosystem continues to mature, with more libraries and tools becoming available to simplify web development tasks.
Rust’s potential to revolutionize web development lies in its ability to provide developers with the confidence to write high-performance code without the fear of common bugs that plague systems programming. This assurance is invaluable in an era where web services require robust security and efficiency.
For those considering Rust, there’s never been a better time to dive in. The community is welcoming and supportive, with a wealth of resources for learners. Whether you’re a seasoned developer or just starting, Rust offers a challenging yet rewarding experience.
Embrace the learning curve, and you’ll discover a language that not only enhances your programming skills but also changes the way you think about software development. Rust is more than a language; it’s a new perspective on creating reliable and maintainable web services.
So, take the leap and explore Rust. You might find that it’s the perfect tool for your next web project, opening doors to opportunities that were previously unattainable. Rust is not just the future; it’s the present, and it’s here to stay in the web development landscape.